Chapter 4: Interpolation and update operations¶
4.1 Interpolation¶
4.1.1 Main procedures involved¶
Agrif_Set_BcInterp() : Type of interpolation at boundaries
Agrif_Set_Bc() : Where to interpolate
Agrif_Bc_Variable() : Make a boundary interpolation
Agrif_Set_Interp() : Type of interpolation within the domain
Agrif_Interp_Variable() : Make an interior interpolation
Agrif_Init_Variable() : To interpolate over the whole domain (within the domain and boundaries)
4.1.2 Interpolation: how to specify where to interpolate ?¶
1. Agrif_Set_Bc¶
Agrif_Set_Bc(variable_id,point)
with point = (/begin,end/)
Examples:
Centered variables
point=(/0,0/)

point=(/-1,-1/)

point=(/0,1/)

Location specification in case of interpolation of centered variable (T) in 1D
Noncentered variables:
point=(/0,0/)

point=(/-1,-1/)

point=(/0,1/)

Location specification in case of interpolation of Noncentered variable (U) in 1D
2. Agrif_Set_BcInterp¶
Agrif_Set_BcInterp(Variable_id,interp=Agrif_Interp_Type)
The default value of agrif_interp_type is agrif_constant .
Interpolation schemes
Order | Conservative | Monotone | |
---|---|---|---|
Agrif_constant | 0 | X | X |
Agrif_linear | 1 | ||
Agrif_linear_conserv | 1 | X | |
Agrif_linear_conservlim | 1 | X | X |
Agrif_lagrange | 2 | ||
Agrif_ppm | 2 | X | X |
Agrif_eno | 2 | X | |
Agrif_weno | 3 | X |
Examples:
1D
Call Agrif_Set_BcInterp(u_id,interp = Agrif_linear)
2D
Call Agrif_Set_BcInterp(u_id,interp = Agrif_linear)
or
Call Agrif_Set_BcInterp(u_id,interp2 = Agrif_PPM)
or
Call Agrif_Set_BcInterp(u_id, interp1 = Agrif_PPM, interp2 = Agrif_PPM)
Note
- Interp1 indicates interpolation in the first dimension
- Interp2 indicates interpolation in the second dimension
4.1.3 The call: Agrif_Bc_Variable¶
1. Agrif_Bc_Variable¶
Agrif_Bc_Variable(variable_id,procname)
Example: procname Interp_MyTraceur
Call Agrif_Bc_Variable(u_id,procname=Interp_MyTraceur)
Algorithm of procname’s call
- AGRIF calls procname on the coarse grid with before = .TRUE. output array : tabres
- AGRIF interpolates tabres on the fine grid
- AGRIF calls procname on the fine grid with before = .FALSE. input array : tabres
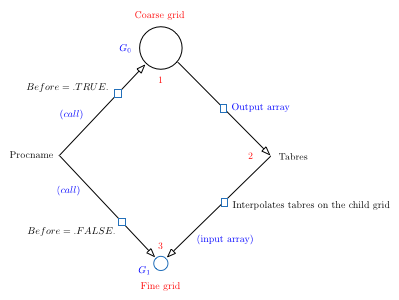
Algorithm of procname’s call by AGRIF for interpolation
Subroutine Interp_My_Traceur(tabres,i1,i2,before)
use module_My_traceur
real,dimension(i1:i2),intent(out) :: tabres
Logical :: before
if (before) then ! on parent grid
tabres(i1:i2) = My_traceur(i1:i2)
else ! on child grid
My_traceur(i1:i2) = tabres(i1:i2)
endif
End Subroutine Interp_MyTraceur
4.1.4 Time interpolation¶
1. How are time interpolations handle ?¶
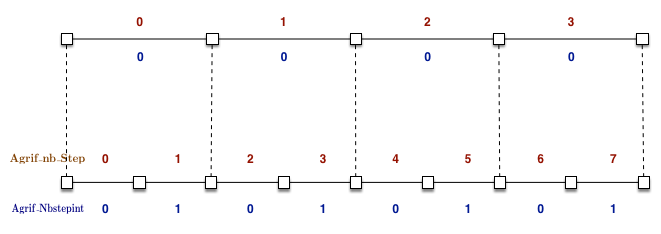
Grid step counters Agrif_Nb_Step() and Agrif_Nbstepint() with a time refinement factor of 2
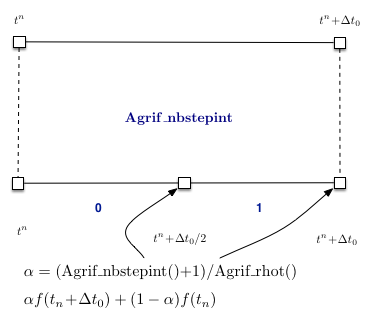
Description of time interpolation
2. Agrif_Bc_Variable¶
Agrif_Bc_Variable (variable_id,procname,calledweight= \(\alpha\))
Inside AGRIF, the spatial interpolation is done only when the time index of the parent grid changes. So, the spatial interpolation is done at the first call of Agrif_Bc_Variable inside a parent grid time step.
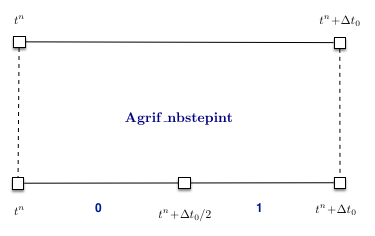
Description of Agrif_Nbstepint
Note
- In order to interpolate the very first parent field, a call to Agrif_Bc_Variable() in the
Agrif_Initvalues
procedure is mandatory. - To force an interpolation (even if the parent grid index has not changed)
Call Agrif_Set_Bc(variable_id,point,Interpolationshouldbemade=.TRUE.)
4.1.5 Interpolation over the whole domain¶
The interpolation over the whole domain is done through the subroutine Agrif_Init_Variable() .
In fact, Agrif_Init_Variable() represents an addition of interpolation within the domain made through a call to the subroutine Agrif_Interp_Variable() and interpolation at boundaries made through a call to Agrif_Bc_Variable() . Interpolation with the subroutine Agrif_Interp_Variable() is made from the first point within the domain to the last point within the domain.
It is important to notice that for boundary interpolation we use the subroutine Agrif_Set_bcinterp to indicate the type of interpolation and the subroutine Agrif_Set_interp() in case of interpolation within the domain. All those two subroutines use the same arguments.
Agrif_Init_Variable() = call to Agrif_Interp_Variable() + call to Agrif_Bc_Variable()
Example :
- Centered points (T):

Interpolation of centered point (T)
- NonCentered points (U):

Interpolation of non-centered point (U)
4.1.6 Treatment of masked fields¶
Agrif_SpecialValue: replacement of masked values by neighboring values.
It must be set before the call to Agrif_Bc_Variable() , Agrif_Interp_Variable() .
Agrif_UseSpecialValue= .true.
Agrif_SpecialValue = Val
Examples:
Agrif_UseSpecialValue=.true.
Agrif_SpecialValue=0.
Call Agrif_Bc_Variable(variable_Id,procname)
Agrif_UseSpecialValue=.false.
Note
Setting maximum lookup of masked values (since it may affect performance).
Call Agrif_Set_MaskMaxSearch ( mymaxsearch )
Defaut Value: MaxSearch = 5
4.2 Updates¶
4.2.1 Main procedures involved¶
Agrif_Set_UpdateType() : Type of update
Agrif_Update_Variable() : Make the restriction and specify where to update
4.2.2 Update: how to specify the type of update ?¶
The type of update has to be indicated through the subroutine Agrif_Set_UpdateType().
1. Agrif_Set_UpdateType¶
Agrif_Set_UpdateType(variable_id,update = Agrif_Update_Type)
The keyword Agrif_Update_Type should be either Agrif_Update_Copy, Agrif_Update_Average or Agrif_Update_Full_Weighting .
2. Update schemes¶
Examples:
1D
Call Agrif_Set_UpdateType(variable_id,update = Agrif_average)
2D
Call Agrif_Set_UpdateType(variable_id,update = Agrif_average) ! same update scheme in all directions
Call Agrif_Set_UpdateType(variable_id,update1 = Agrif_Copy, update2 = Agrif_Average)
Example with the space refinement of 3
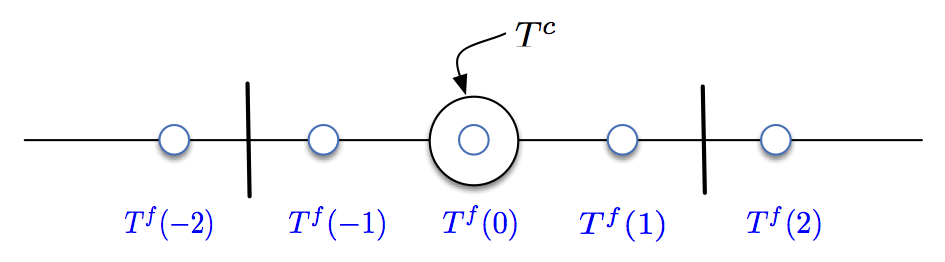
Relative positions of coarse and fine points with space refinement of 3
copy: \(T^c = T^f(0)\)
Average: \(T^c = \frac{1}{3}[T^f(-1)+T^f(0)+T^f(1)]\)
FW: \(T^c = \frac{1}{9}[T^f(-2)+2T^f(-1)+3T^f(0)+2T^f(1)+T^f(2)]\)
4.2.3 The call: Agrif_Update_Variable¶
Agrif_Update_Variable(variable_id,locupdate,procname)
Example:
Call Agrif_Update_Variable(u_id,locupdate=(/0,0/), procname=Update_MyTraceur)
Note
- locupdate is an optional keyword that indicates the location where the update takes place.
4.2.4 Specify locations you want to update¶
1. Without locupdate keyword¶
Agrif_Update_Variable(variable_id,procname)
Note
Default location without locupdate keyword is interior of the reference grid
Examples:
- Centered variable:

Update the centered variable without locupdate keyword
- Noncentered variable:

Update the Noncentered variable without locupdate keyword
2. With locupdate keyword¶
Agrif_Update_Variable(variable_id,locupdate,procname)
Note
Update is done on limited areas
- For multidimension we set the keyword as:
locupdate1=... , locupdate2 = ...
Examples
- Centered points:
locupdate=(/0,0/)

locupdate=(/1,1/)

locupdate=(/0,1/)

locupdate=(/1,0/)

Update of centered points(T) with locupdate keyword
- Non centered points:
locupdate=(/0,0/)

locupdate=(/1,1/)

locupdate=(/0,1/)

locupdate=(/1,0/)

Update of non-centered points (U) with locupdate keyword
4.2.5 The use of procnames¶
1. Agrif_Update_Variable¶
Agrif_Update_Variable(variable_id,procname)
Call Agrif_Update_Variable(variable_id,procname=Update_MyTraceur)
Algorithm of procname’s call
- AGRIF calls procname on the child grid with before = .TRUE. output array : tabres
- AGRIF updates tabres on the parent grid
- AGRIF calls procname on the coarse grid with before = .FALSE. input array : tabres
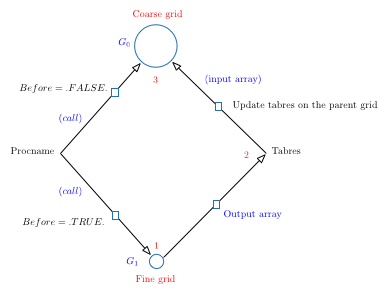
Algorithm of procname’s call by AGRIF for update
Subroutine Update_My_Traceur(tabres,i1,i2,before)
use module_My_traceur
real,dimension(i1:i2),intent(inout) :: tabres
logical :: before
If (before) then ! on the child grid
tabres(i1:i2) = My_traceur(i1:i2)
Else ! on the parent grid
My_traceur(i1:i2) = tabres(i1:i2)
Endif
End Subroutine Update_My_Traceur
4.2.6 Treatment of masked fields¶
Agrif_SpecialValue_InfineGrid: the masked values are not taken into account in the restriction operation
Agrif_UseSpecialValueInUpdate = .true.
Agrif_SpecialValueFineGrid = Val
must be set before the call to Agrif_Update_Variable()
Examples:
Agrif_UseSpecialValueInUpdate=.true.
Agrif_SpecialValueFineGrid=0.
Call Agrif_Update_Variable(variable_id,procname=Update_MyTraceur)
Agrif_UseSpecialValueInUpdate=.false.