Chapter 2: General organization of an AGRIF code¶
Example: Main program
program example
use mod_global
integer :: i
call Agrif_Init_Grids()
call read_config()
call init() ! initialize the coarse grid
do i=1,nbsteps
call Agrif_Step(step) ! is used to integrate the grid hierarchy which is created at the first call
enddo
end program example
2.1 Main AGRIF procedures¶
They have to be called:
Agrif_Init_Grids() : very first call in the code: initializes Agrif library.
Agrif_Step(step) : integrates the model forward on all grids by calling step() recursively.
They can be called:
Agrif_Regrid() : reads AGRIF_Fixed_Grids.in and build grids data structures (called anyway if not explicitly).
Agrif_Step(step) : calls step() recursively on each grid (with time refinement).
Agrif_Step_Child(step) : calls step() recursively on each grid (no time refinement).
Agrif_Step_Childs(step) : calls step() recursively on each child grid of the current Parent grid (no time refinement).
Agrif_Integrate_ChildGrids(step) : calls step() recursively on each child grid of the current Parent grid (with time refinement).
They have to be written by the user:
Agrif_InitWorkspace(): which defines dimensions of the current workspace. It is Called each time the library needs to change the current grid.
Example: Agrif_InitWorkspace
subroutine Agrif_InitWorkspace ()
use mod_global
! compute everything that is related to array sizes
Nnx = nx + 1
Nny = ny + 1
end subroutine Agrif_InitWorkspace
Agrif_InitValues: which is the initialization routine called once for each fine grid. Typically it is used to:
- declare Agrif profiles for interpolation/restriction operations
- initialize variables (eg: read data from file / interpolation from coarse grid)
Example: Agrif_InitValues()
subroutine Agrif_InitValues()
call init()
end subroutine Agrif_InitValues
Note
The first call of Agrif_Step() calls Agrif_Regrid() and Agrif_InitValues() on the whole grids and initializes all.
2.2 Integration of the grids hierarchy¶
There are four subroutines that involve the integration of grid hierarchy: Agrif_Step(), Agrif_Step_Child(), Agrif_Step_Childs() and Agrif_Integrate_ChildGrids() .
2.2.1 Agrif_Step(step)¶
It calls step() recursively on each grid and takes into account the time refinementfactor.
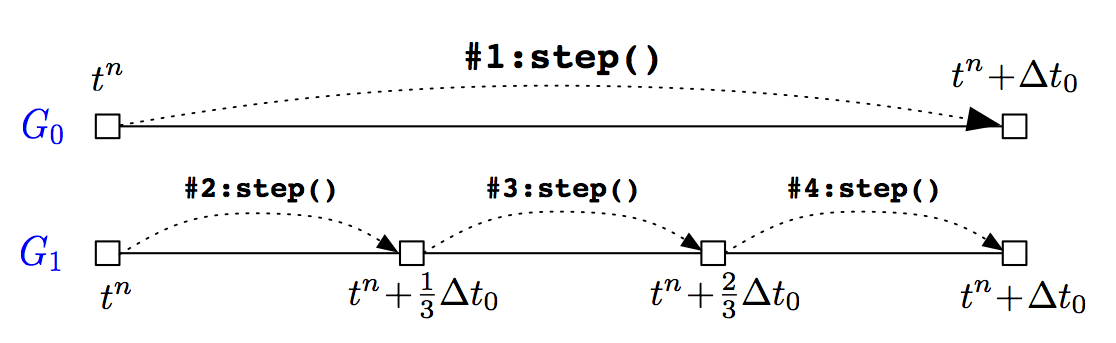
Description Agrif_Step subroutine with a root grid G0 and a child grid G1 (Time refinement factor = 3)
2.2.2 Agrif_Step_Child(step)¶
It calls step() recursively on each grid (no time refinement). This subroutine could be used instead of Agrif_Step(step) in order to obtain the same result. In fact, we introduce a call of Agrif_Step_Child(step) at the end of subroutine step().
Example: subroutine step()
subroutine step()
. .
. .
. .
call agrif_step_child(step)
call agrif_update_variable(u_id,procname=Update_MyTraceur)
end subroutine step
2.2.3 Agrif_Step_Childs(step)¶
It calls step() recursively on each child grid of the current Parent grid (no time refinement).
2.2.4 Agrif_Integrate_ChildGrids(step)¶
It calls step() recursively on each child grid of the current Parent grid (with time refinement). The difference with Agrif_Step(step) is the fact that step() is called only on the childs grids not on the parent grid.
2.3 Auxiliary Agrif functions¶
Agrif_Root() : indicates if the current grid is the root grid
Agrif_Fixed() : returns the number of the current grid (0 for root)
Agrif_IRhox() : returns the space refinement factor of the current grid
Agrif_IRhot() : returns the time refinement factor of the current grid
Agrif_Nb_Step() : number time steps for current grid
Agrif_Nbstepint() : sub-step number inside parent grid integration
Agrif_Parent(X) : gets the value of X on the parent grid where X is a scalar variable
Example: Set the time for update by using Agrif’s functions
if (two_way.and.(Agrif_Nbstepint() == Agrif_IRhot()-1) ) then
call Agrif_Update_Variable(Variable_id,procname = update_MyTraceur)
endif